Working with JSON
Several built-in processor support dynamic types such as maps/dictionaries and lists/collections.
The secret to processing JSON in Templater is converting JSON input into such dynamic types.
Many Java libraries do that by default, while in .NET
additional conversion
is required to get native dictionaries/values.
Example of JSON usage through Console application can be found among
Github examples.
Main benefit of such project is to easily learn/explore how Templater behaves through template and JSON
tweaking by looking at the output result after running the console application.
An example Invoice JSON
{
"number": 1234,
"date": "today",
"paid": true,
"customer": {
"name": "Satisfied customer Ltd",
"address": "Worldwide"
},
"items": [
{"product": "Templater license", "quantity": 2, "price": 199.00},
{"product": "Documentation", "quantity": 1, "price": 0.00}
]
}
Will be converted into appropriate maps, lists and values (numbers, booleans, strings,...)
Map(
number: Integer(1234),
date: String(today),
paid: Boolean(true),
customer: Map(
name: String(Satisfied customer Ltd),
address: String(Worldwide)
),
items: List(
Map(product: String(Templater license), quantity: Integer(2), price: Decimal(199.00)),
Map(product: String(Documentation), quantity: Integer(1), price: Decimal(0.00))
)
)
Templater will process it by matching relevant tags:
- [[number]]
- [[date]]
- [[paid]]
- [[customer.name]]
- [[customer.address]]
- [[items.product]]
- [[items.quantity]]
- [[items.price]]
Major distinction from other processing solutions is that there is no for loop for processing items. There is only navigation over its properties. This works by Templater matching relevant [[items. tags in the document and duplicating it depending on the number of elements in a list. For template which looks like:
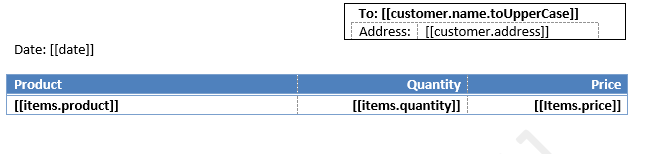
After Templater matches items intermediary document/template will look like:
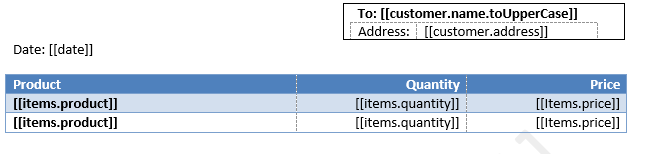
Templater will now start processing each element in the list, so appropriate values will end up in the expected position:
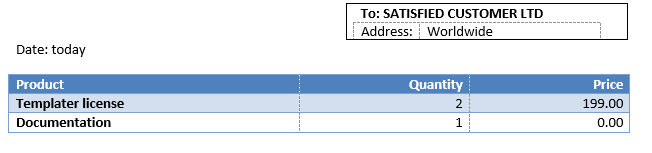
By combining metadata and plugins more complex scenarios can be supported with JSON,
such as inserting images into the document.
The prebuilt executables for processing JSON
also support image conversion from
base64 input strings.
This way tag like: [[logo]:image] when matched against relevant JSON:
{
"logo": "iVBORw0KGgoAAAANSUhEUgAAAGQAAABDCAMAAABdlVDoAAADAFBMVEUsNzctNzguODguODkvOTowOjowOjsxOzwyPDwzPD00PT00Pj41Pj82P0A3QEE4QUE4QUI5QkM7Q0Q7REQ7REU8REU9RUY+Rkc/R0g/SEhAR0hASEhASUlBSUpCSkpCSktES0xETExFTU1GTk5HTk9IT09IUFBKUVFKUlJMU1NMVFROVVVPVlVQV1ZQV1dRWFdRWFhSWVlTWllUWlpUW1tVXFtWXFxWXV1ZYWFhaWliaWpiampja2tka2tlbGxlbW1mbW5nbm5obm5obm9ob3BpcHBpcHFrcXJrcnJscnNsc3RudHRudHVvdXZwdnZwdndyeHhzeXl0enp1ent2fHx3fX14fX15fn55f396gH8SgMQwkMt6gIB8gYB8gYF9goF9goJ9g4N/hIRNoNOAhYWLkZKnq6uoq6uorKyIv+G9wMDKzc3Lzc7Lzs7Mzs7Mz8/Nz9DN0NDO0NHQ0tLR09PS1NTT1dXU1dXU1tbY2trE3/DS5/Tp6urh7/j///8AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAADdRLlPAAAACXBIWXMAAA7EAAAOxAGVKw4bAAADkklEQVR42u2YV1cTURSFj0oUbKAgiSUBMQkpkwYBe1csoFEBQcVgbyhKSIJ1frvnlrmZe5YpBx9cy8V+0W+d7P0NZV4At11+vGyTtgsutP3E98dtsiXZkvxTyYc3b9/pvH3z0yXYsaTlDJRzhcLYOGaskM/VXYMFifbL+KSxTF/GZjMSYTmPVMQIrrsE7bxv/j1qOQPLhcJ4cWJycqKID7/hPhNHDxkSq0dmNgAR6eRJ5DF5HVconmGDJfnjzISYgQaiVF2RvA8zJNizJY0ZaPkIPMkfZ6TE+5l89X1vJw12LJF5ZX4mNprfLr0qncWJ4qYkL+zfSoPmPdGr5byFPMlz+WIUcnU/iveknEVLwXyJ5Wzej0yJaOZz2TpBeJrN5vJ5syrxLyR5HPVJBGbqsORkcDfnfYlLTtaPPMkyFrPZjFMjCI/STgY1nn3JRp6kjMVMxknXfOggwmIqnU47jmcX6DSQJ1nCIq6lagRhIYXB/+oDQaZETKZSyRpBeJhMJsWwPhDkSRZFM5lM1AjCfCKRwGHPTpAnWRBNHKgRhLnRhIw+EORJ5lV3tEoQ5uLx+ChGH+YUeciUiCbuVQnCbCwuow+zcQt5kjnVjVUJwoNoNBaLmVWCbAmWo9EqQXhwAhM1B4I8ySwWRb9KEO6PYBoHgjyJeELRXycI94+LmANBpmRkRNbXCcK9oeHh4caBIE8inhDrQ+sEoRSJDInoQ0nR0KYk92Q1ElknCKVj4XAkYg6lsIU8SUk0w+FjFYIoUfEONjIlTbbgTujIURF9IMiT3JXVI6GKH0OhCswEgyERfSDIk9yR1WCwQhCmBweDIvowrSi4KcmMrA4OVggKiYyRWMiTNNuC2wMDh0TW1IEgT3JbVgcG1gjCrYOY/v5+fSDIk9zCouivEYQbB1T0gSBPctMuG4SpPusw9TcS/YR9XwjCVG+fjD4Q5EmmVLf3sx97ez/D9f0q3sFGpqTJFlzbp6IPBHmSZltwda+KPhDkSZptweU9KvpAkCe5YpcNwqWenp7dmFV1IMiTXBZNHFglCBe7u3tETl3EfHMJdix5LT5/WFa7u1cJwoWdmF0Y8e8nl2DHkpYzcD6w0yTwyfVhIMCQNJ9BhHNdAZOujy7BjiUtZ+DsDl9WXD9uX+lc0nIGzmz3Ba82diwhMzbCafBlxVW4zcOOJaRnY/s/DzLek83/DXJLsiX5fyS/vpuwJL8BByfOYx+m+94AAAAASUVORK5CYII="
}
Will result in the appropriate image
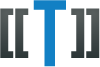